Forms
Toggle buttons
Introduction
The toggle buttons input provides a group of buttons for selecting a single value, or multiple values, from a list of predefined options:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('status')
->options([
'draft' => 'Draft',
'scheduled' => 'Scheduled',
'published' => 'Published'
])
As well as allowing a static array, the options()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Field | Filament\Forms\Components\Field | $component | The current field component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current form data. Validation is not run. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Raw state | mixed | $rawState | The current value of the field, before state casts were applied. Validation is not run. |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the field. Validation is not run. |
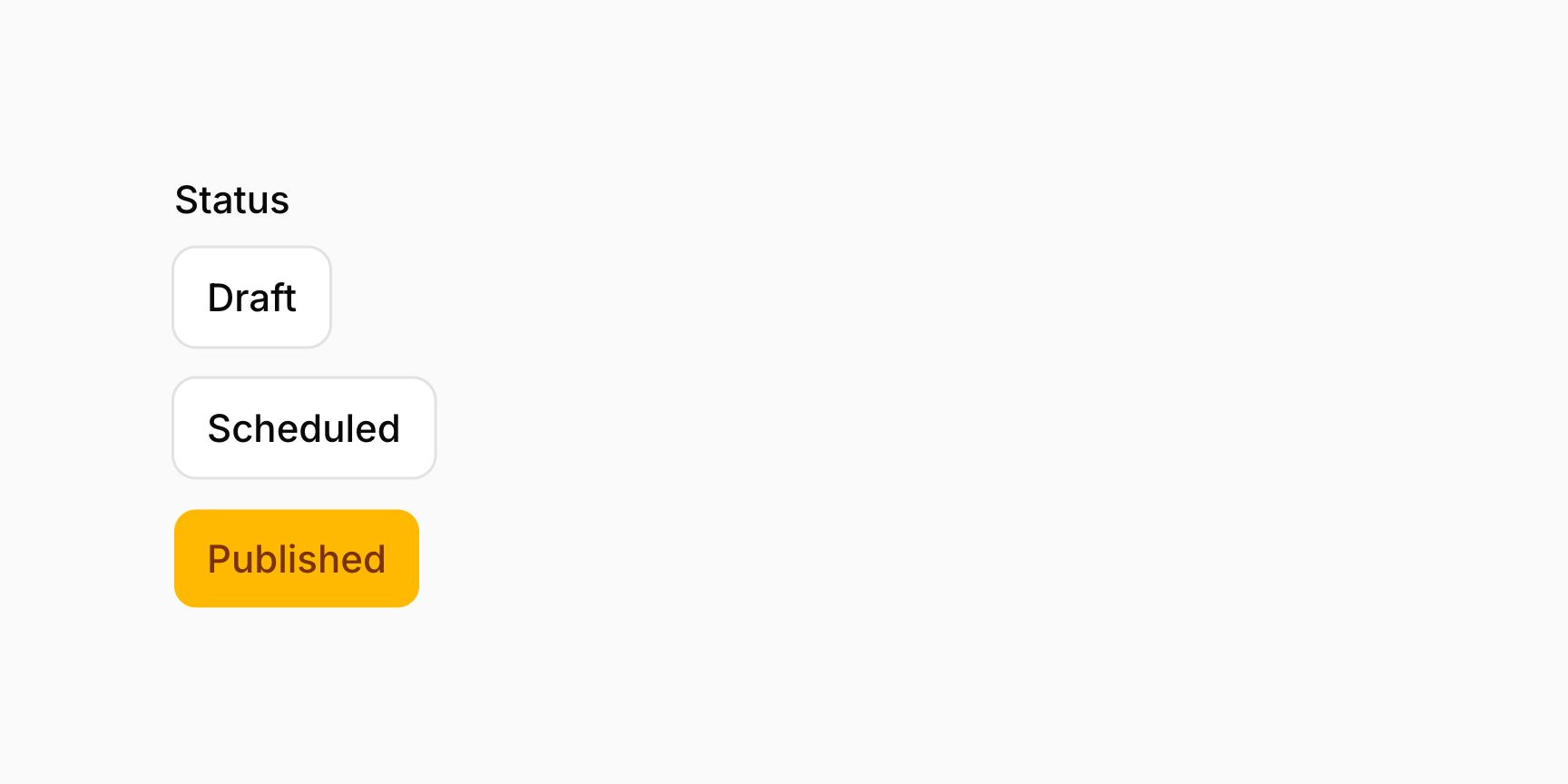
Changing the color of option buttons
You can change the color of the option buttons using the colors()
method. Each key in the array should correspond to an option value:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('status')
->options([
'draft' => 'Draft',
'scheduled' => 'Scheduled',
'published' => 'Published'
])
->colors([
'draft' => 'info',
'scheduled' => 'warning',
'published' => 'success',
])
If you are using an enum for the options, you can use the HasColor
interface to define colors instead.
As well as allowing a static array, the colors()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Field | Filament\Forms\Components\Field | $component | The current field component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current form data. Validation is not run. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Raw state | mixed | $rawState | The current value of the field, before state casts were applied. Validation is not run. |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the field. Validation is not run. |
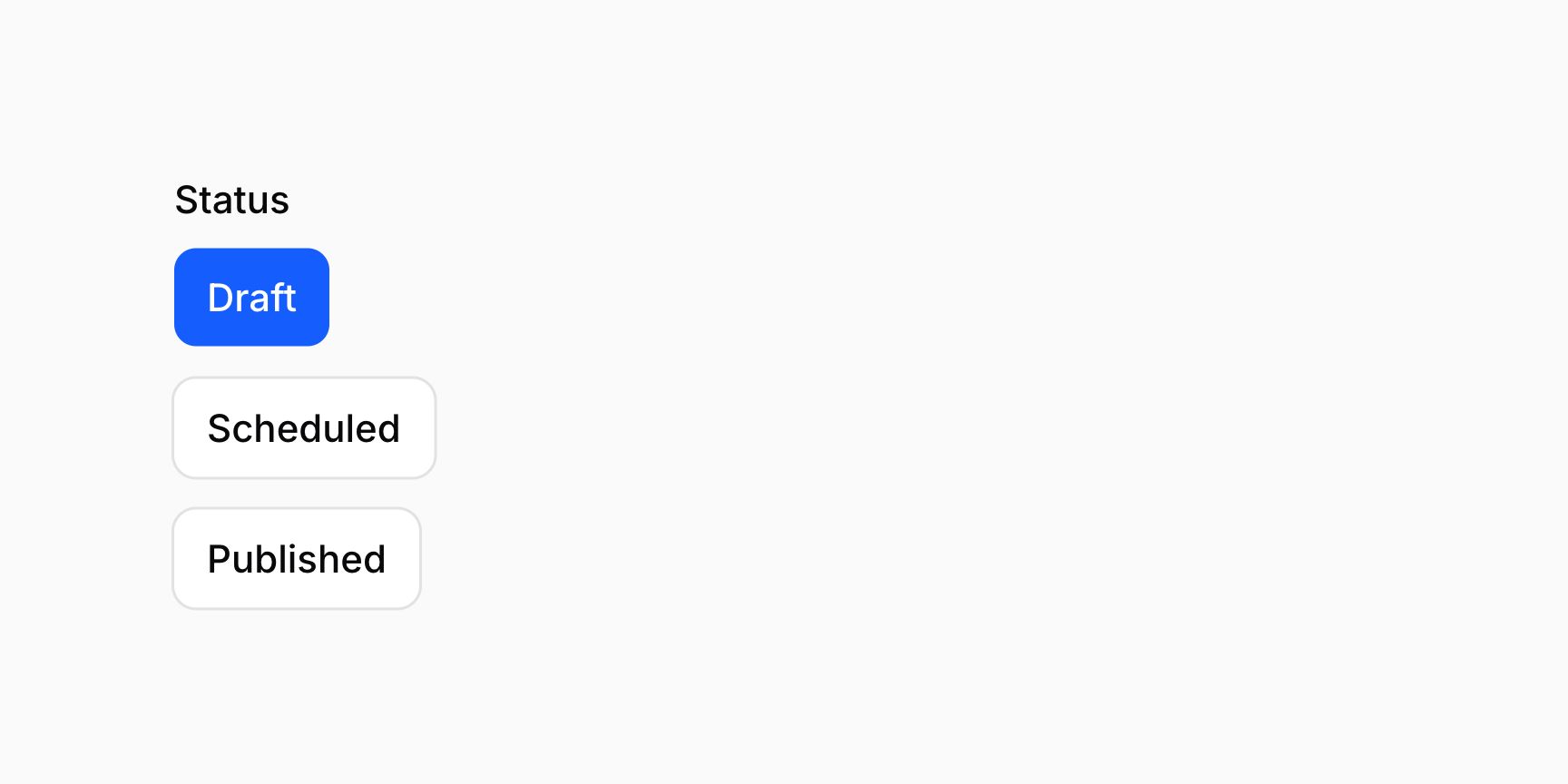
Adding icons to option buttons
You can add icon to the option buttons using the icons()
method. Each key in the array should correspond to an option value, and the value may be any valid icon:
use Filament\Forms\Components\ToggleButtons;
use Filament\Support\Icons\Heroicon;
ToggleButtons::make('status')
->options([
'draft' => 'Draft',
'scheduled' => 'Scheduled',
'published' => 'Published'
])
->icons([
'draft' => Heroicon::OutlinedPencil,
'scheduled' => Heroicon::OutlinedClock,
'published' => Heroicon::OutlinedCheckCircle,
])
As well as allowing a static array, the icons()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Field | Filament\Forms\Components\Field | $component | The current field component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current form data. Validation is not run. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Raw state | mixed | $rawState | The current value of the field, before state casts were applied. Validation is not run. |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the field. Validation is not run. |
If you are using an enum for the options, you can use the HasIcon
interface to define icons instead.
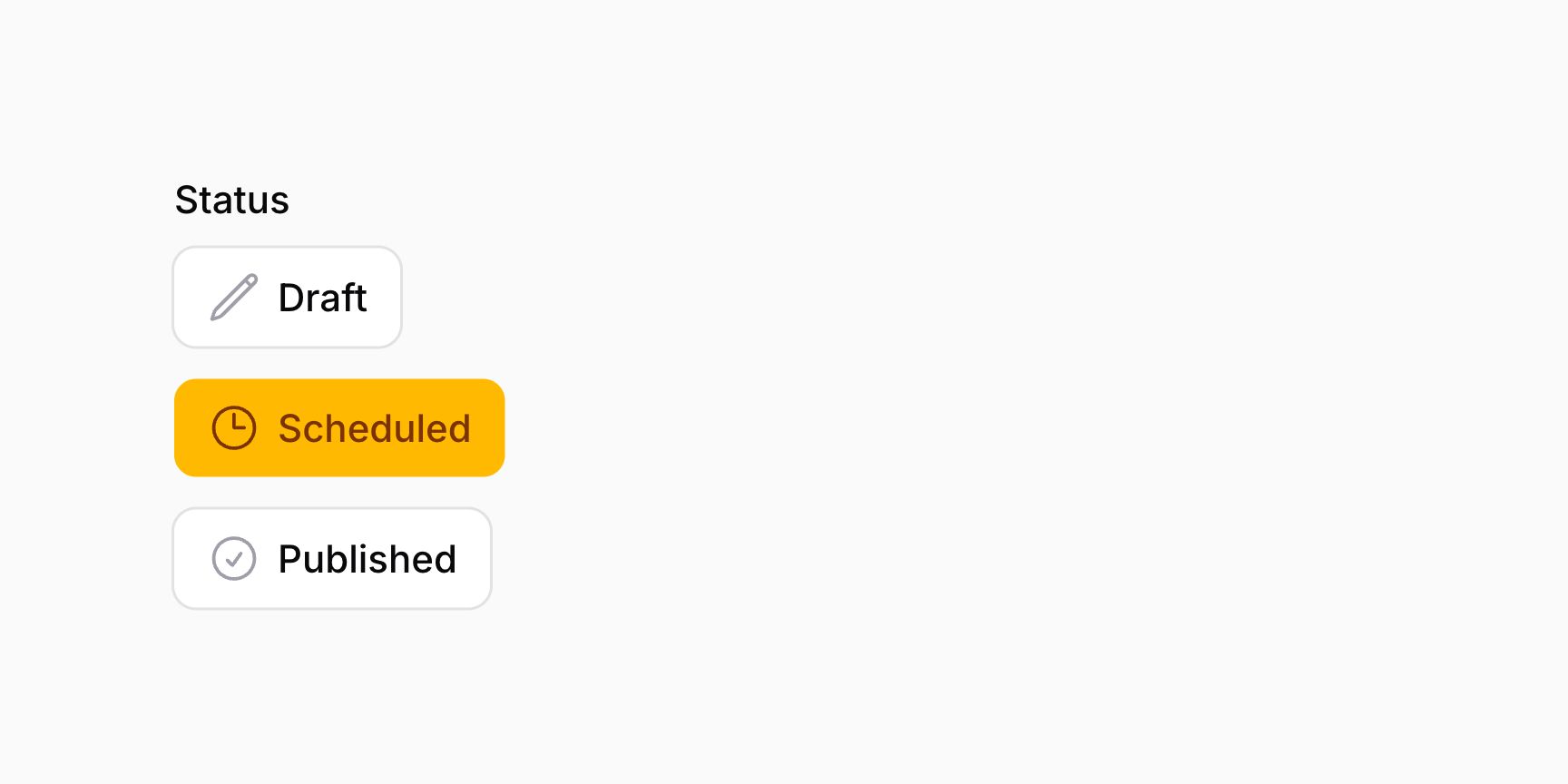
If you want to display only icons, you can use hiddenButtonLabels()
to hide the option labels.
Boolean options
If you want a simple boolean toggle button group, with “Yes” and “No” options, you can use the boolean()
method:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('feedback')
->label('Like this post?')
->boolean()
The options will have colors and icons set up automatically, but you can override these with colors()
or icons()
.
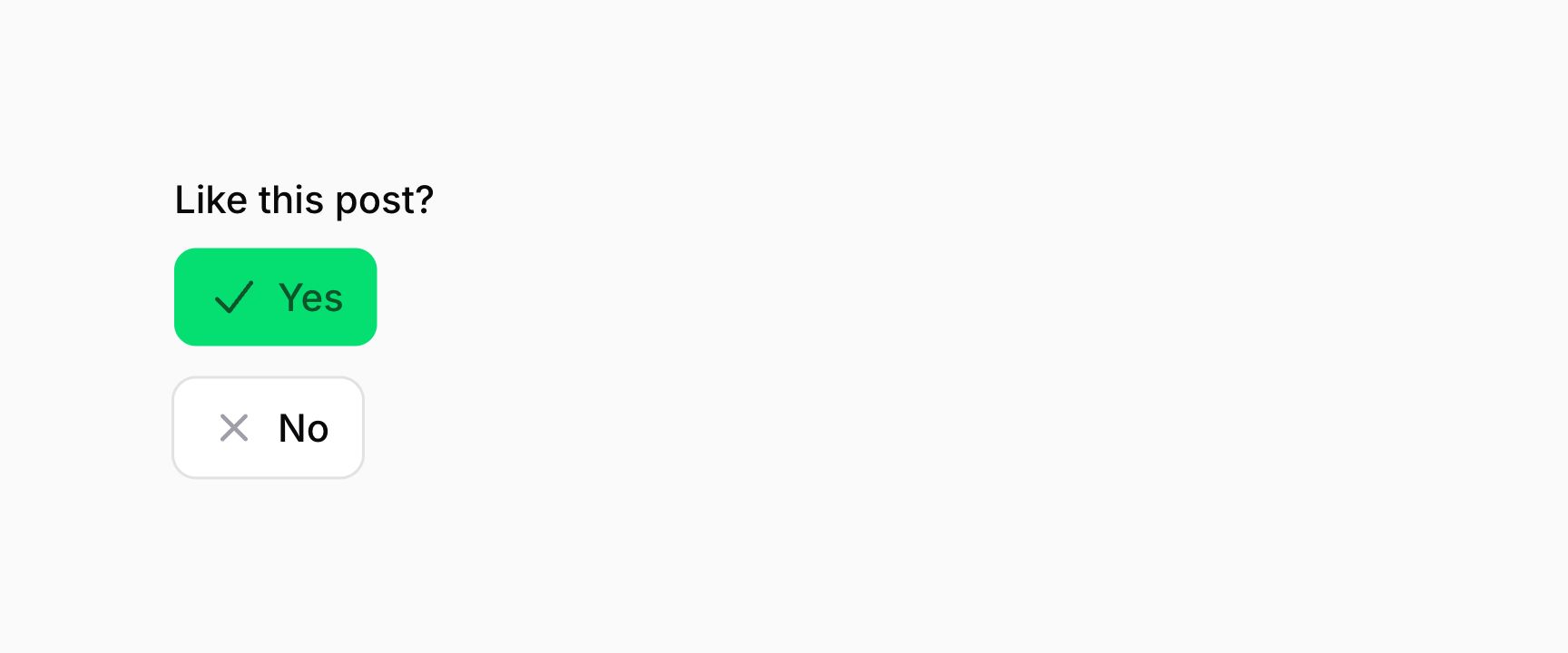
To customize the “Yes” label, you can use the trueLabel
argument on the boolean()
method:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('feedback')
->label('Like this post?')
->boolean(trueLabel: 'Absolutely!')
To customize the “No” label, you can use the falseLabel
argument on the boolean()
method:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('feedback')
->label('Like this post?')
->boolean(falseLabel: 'Not at all!')
Positioning the options inline with each other
You may wish to display the buttons inline()
with each other:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('feedback')
->label('Like this post?')
->boolean()
->inline()
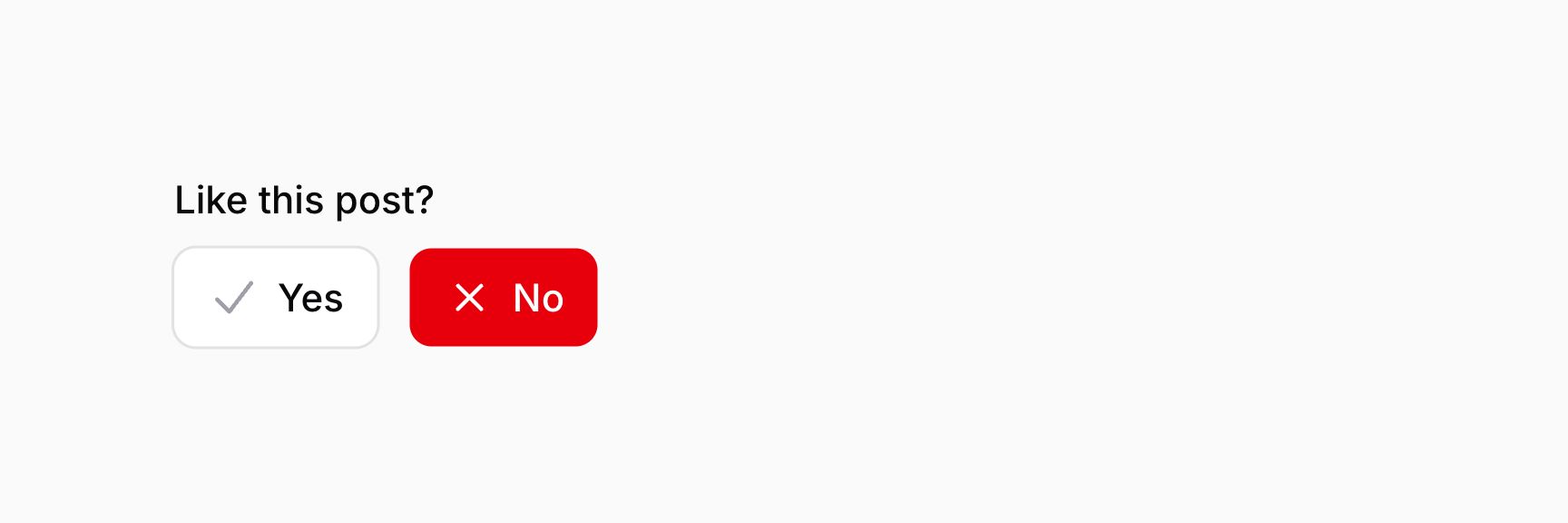
Optionally, you may pass a boolean value to control if the buttons should be inline or not:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('feedback')
->label('Like this post?')
->boolean()
->inline(FeatureFlag::active())
As well as allowing a static value, the inline()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Field | Filament\Forms\Components\Field | $component | The current field component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current form data. Validation is not run. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Raw state | mixed | $rawState | The current value of the field, before state casts were applied. Validation is not run. |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the field. Validation is not run. |
Grouping option buttons
You may wish to group option buttons together so they are more compact, using the grouped()
method. This also makes them appear horizontally inline with each other:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('feedback')
->label('Like this post?')
->boolean()
->grouped()
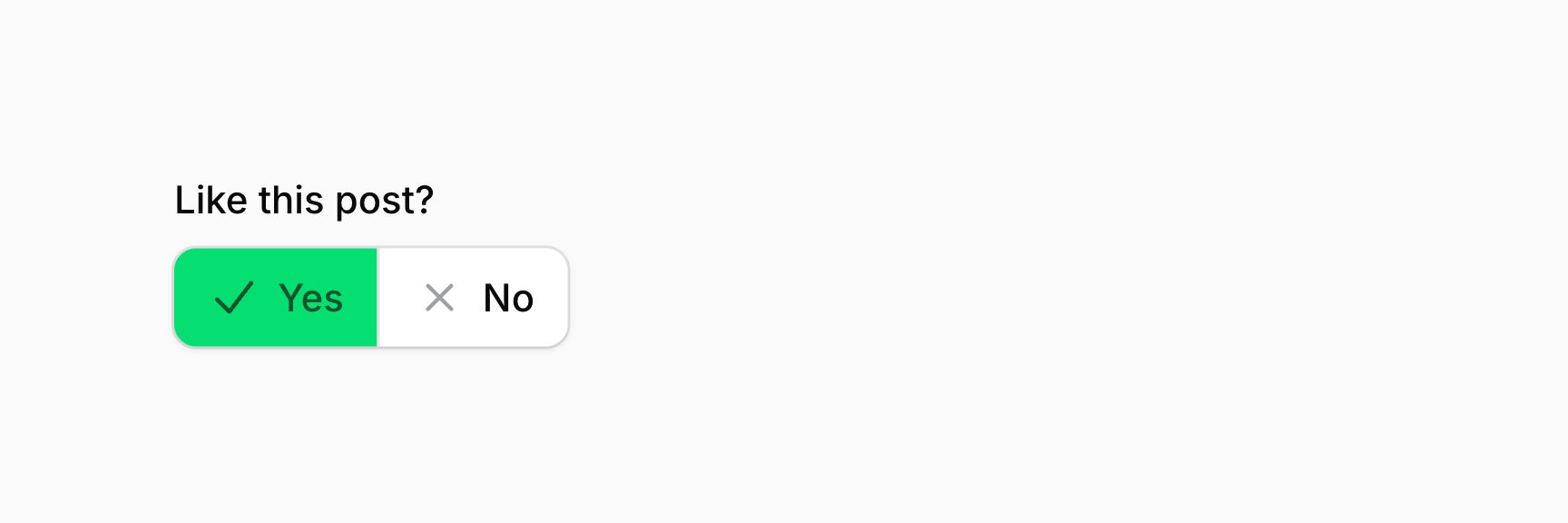
Optionally, you may pass a boolean value to control if the buttons should be grouped or not:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('feedback')
->label('Like this post?')
->boolean()
->grouped(FeatureFlag::active())
As well as allowing a static value, the grouped()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Field | Filament\Forms\Components\Field | $component | The current field component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current form data. Validation is not run. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Raw state | mixed | $rawState | The current value of the field, before state casts were applied. Validation is not run. |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the field. Validation is not run. |
Selecting multiple buttons
The multiple()
method on the ToggleButtons
component allows you to select multiple values from the list of options:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('technologies')
->multiple()
->options([
'tailwind' => 'Tailwind CSS',
'alpine' => 'Alpine.js',
'laravel' => 'Laravel',
'livewire' => 'Laravel Livewire',
])
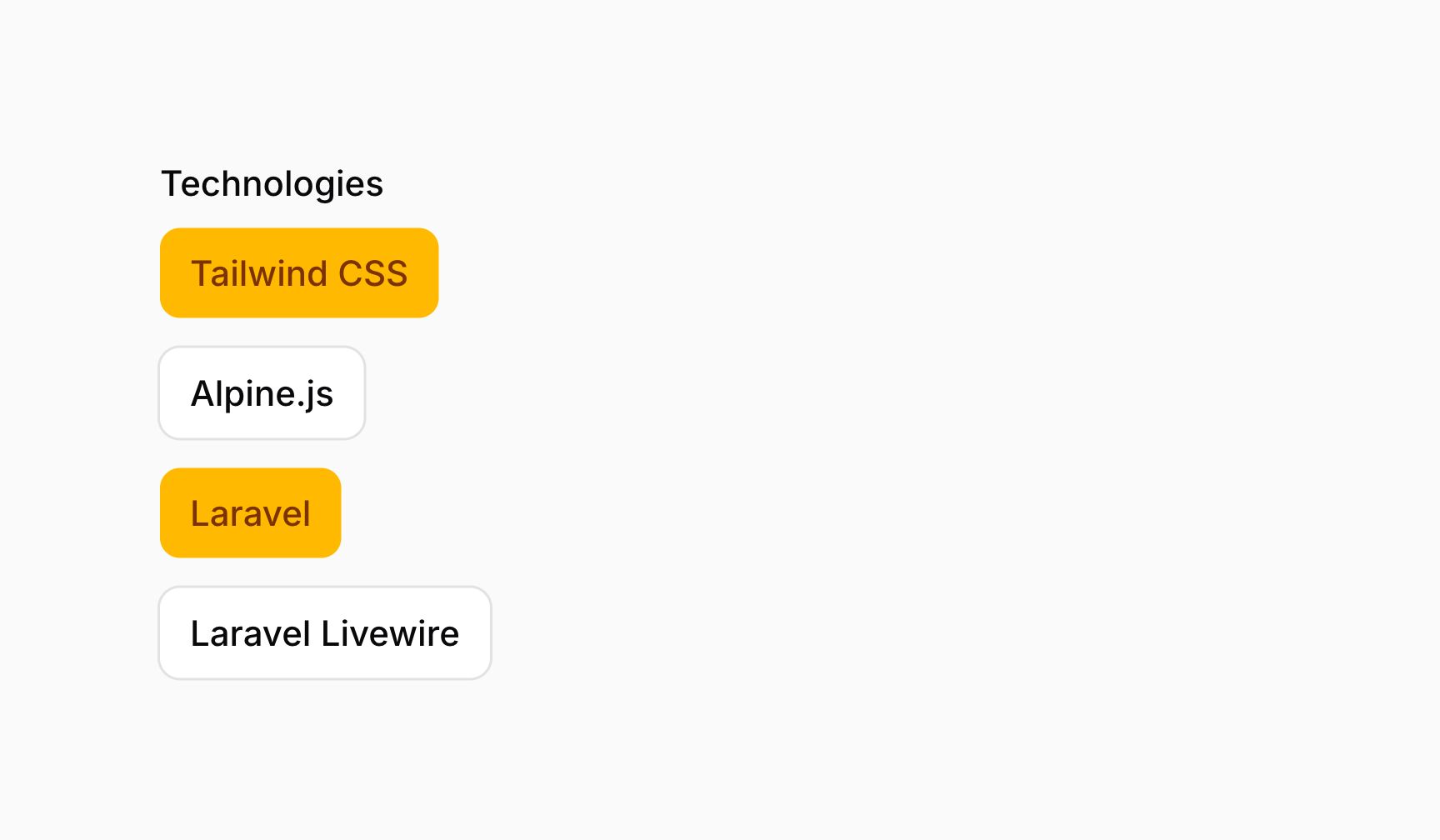
These options are returned in JSON format. If you’re saving them using Eloquent, you should be sure to add an array
cast to the model property:
use Illuminate\Database\Eloquent\Model;
class App extends Model
{
protected $casts = [
'technologies' => 'array',
];
// ...
}
Optionally, you may pass a boolean value to control if the buttons should allow multiple selections or not:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('technologies')
->multiple(FeatureFlag::active())
->options([
'tailwind' => 'Tailwind CSS',
'alpine' => 'Alpine.js',
'laravel' => 'Laravel',
'livewire' => 'Laravel Livewire',
])
As well as allowing a static value, the multiple()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Field | Filament\Forms\Components\Field | $component | The current field component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current form data. Validation is not run. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Raw state | mixed | $rawState | The current value of the field, before state casts were applied. Validation is not run. |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the field. Validation is not run. |
Splitting options into columns
You may split options into columns by using the columns()
method:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('technologies')
->options([
// ...
])
->columns(2)
As well as allowing a static value, the columns()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Field | Filament\Forms\Components\Field | $component | The current field component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current form data. Validation is not run. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Raw state | mixed | $rawState | The current value of the field, before state casts were applied. Validation is not run. |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the field. Validation is not run. |
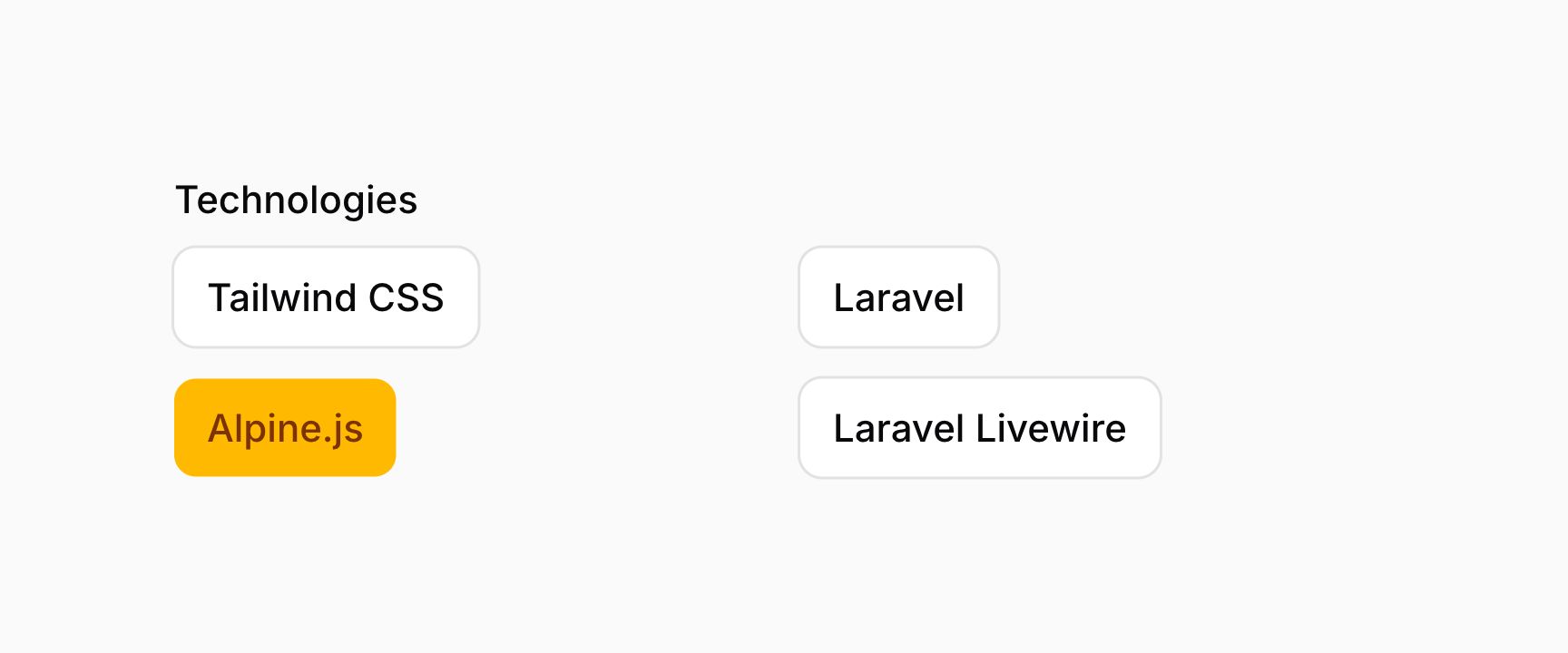
This method accepts the same options as the columns()
method of the grid. This allows you to responsively customize the number of columns at various breakpoints.
Setting the grid direction
By default, when you arrange buttons into columns, they will be listed in order vertically. If you’d like to list them horizontally, you may use the gridDirection(GridDirection::Row)
method:
use Filament\Forms\Components\ToggleButtons;
use Filament\Support\Enums\GridDirection;
ToggleButtons::make('technologies')
->options([
// ...
])
->columns(2)
->gridDirection(GridDirection::Row)
As well as allowing a static value, the gridDirection()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Field | Filament\Forms\Components\Field | $component | The current field component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current form data. Validation is not run. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Raw state | mixed | $rawState | The current value of the field, before state casts were applied. Validation is not run. |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the field. Validation is not run. |
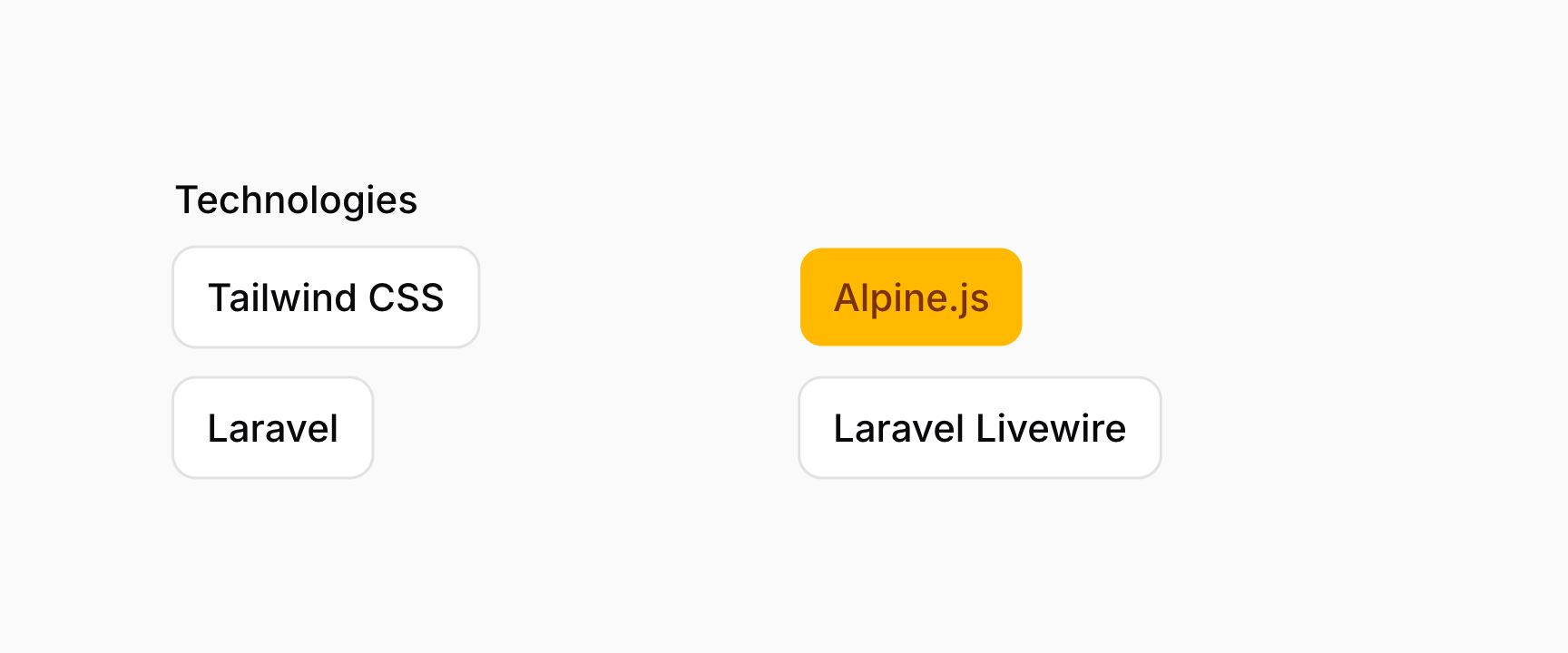
Disabling specific options
You can disable specific options using the disableOptionWhen()
method. It accepts a closure, in which you can check if the option with a specific $value
should be disabled:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('status')
->options([
'draft' => 'Draft',
'scheduled' => 'Scheduled',
'published' => 'Published',
])
->disableOptionWhen(fn (string $value): bool => $value === 'published')
You can inject various utilities into the function as parameters.
Learn more about utility injection.Utility | Type | Parameter | Description |
---|---|---|---|
Field | Filament\Forms\Components\Field | $component | The current field component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current form data. Validation is not run. |
Option label | string | Illuminate\Contracts\Support\Htmlable | $label | The label of the option to disable. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Raw state | mixed | $rawState | The current value of the field, before state casts were applied. Validation is not run. |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the field. Validation is not run. |
Option value | mixed | $value | The value of the option to disable. |
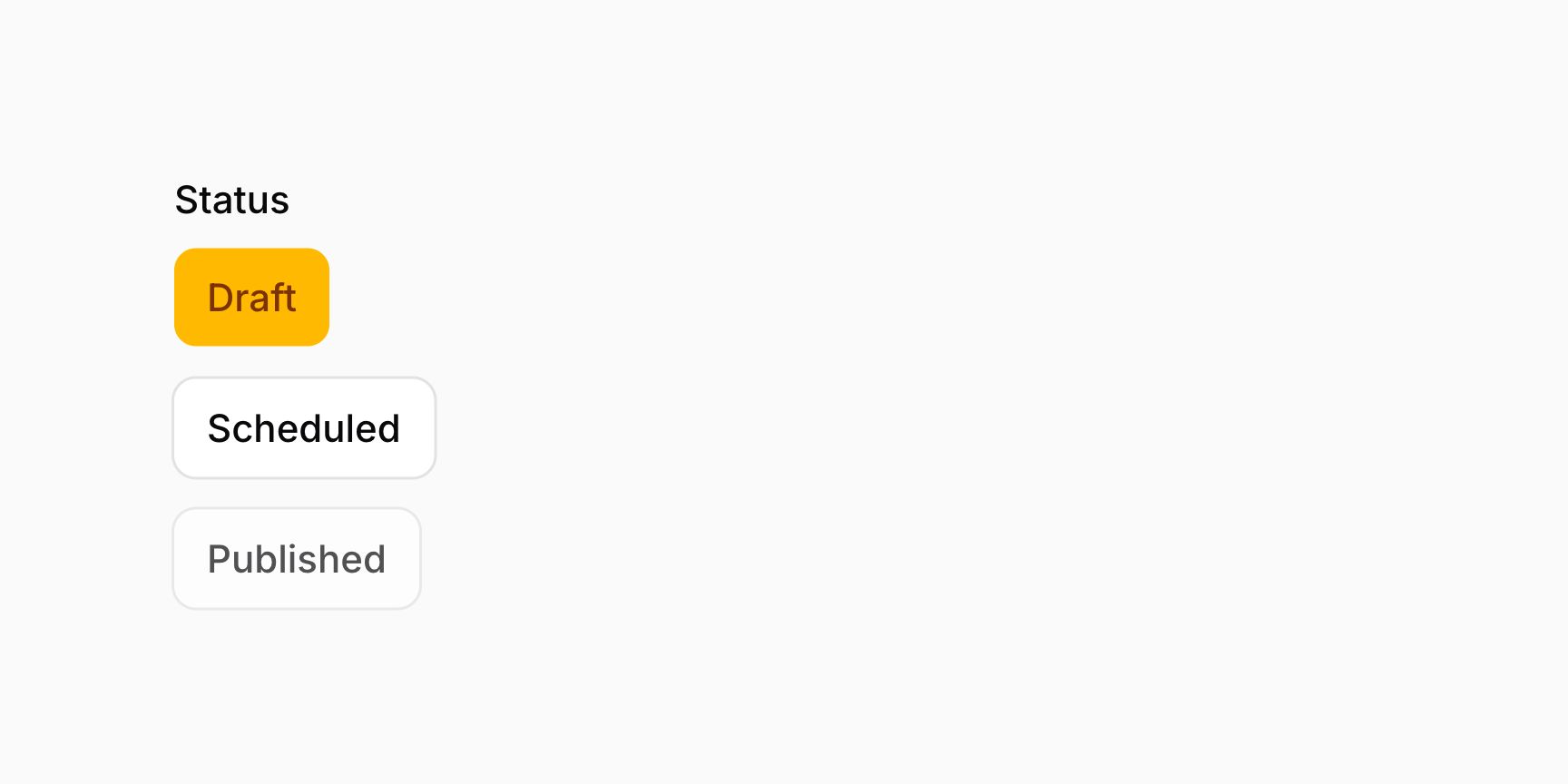
If you want to retrieve the options that have not been disabled, e.g. for validation purposes, you can do so using getEnabledOptions()
:
use Filament\Forms\Components\ToggleButtons;
ToggleButtons::make('status')
->options([
'draft' => 'Draft',
'scheduled' => 'Scheduled',
'published' => 'Published',
])
->disableOptionWhen(fn (string $value): bool => $value === 'published')
->in(fn (ToggleButtons $component): array => array_keys($component->getEnabledOptions()))
For more information about the in()
function, please see the Validation documentation.
Still need help? Join our Discord community or open a GitHub discussion