Infolists
Repeatable entry
Introduction
The repeatable entry allows you to repeat a set of entries and layout components for items in an array or relationship.
use Filament\Infolists\Components\RepeatableEntry;
use Filament\Infolists\Components\TextEntry;
RepeatableEntry::make('comments')
->schema([
TextEntry::make('author.name'),
TextEntry::make('title'),
TextEntry::make('content')
->columnSpan(2),
])
->columns(2)
As you can see, the repeatable entry has an embedded schema()
which gets repeated for each item.
For example, the state of this entry might be represented as:
[
[
'author' => ['name' => 'Jane Doe'],
'title' => 'Wow!',
'content' => 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam euismod, nisl eget aliquam ultricies, nunc nisl aliquet nunc, quis aliquam nisl.',
],
[
'author' => ['name' => 'John Doe'],
'title' => 'This isn\'t working. Help!',
'content' => 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam euismod, nisl eget aliquam ultricies, nunc nisl aliquet nunc, quis aliquam nisl.',
],
]
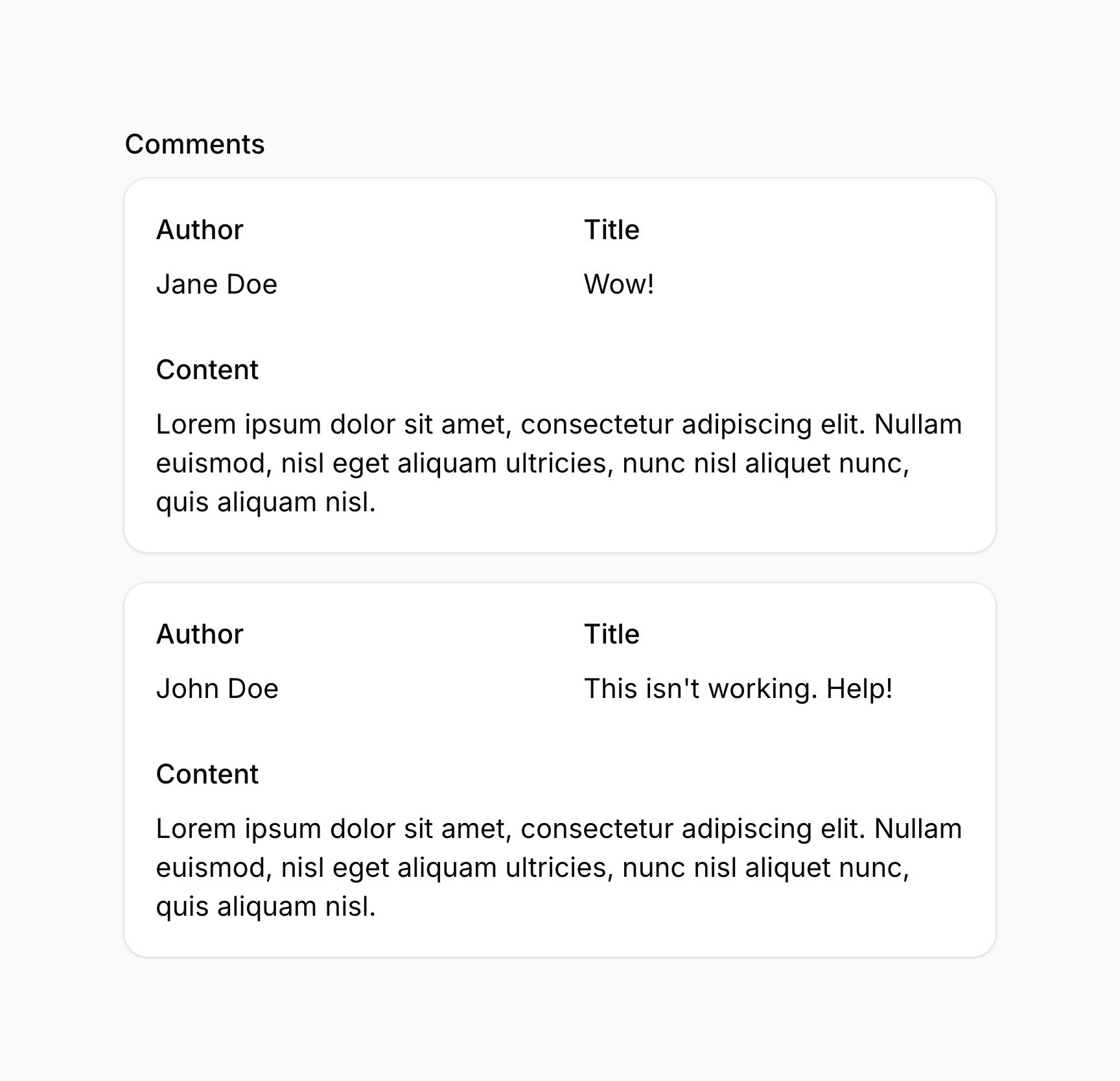
Alternatively, comments
and author
could be Eloquent relationships, title
and content
could be attributes on the comment model, and name
could be an attribute on the author model. Filament will automatically handle the relationship loading and display the data in the same way.
Grid layout
You may organize repeatable items into columns by using the grid()
method:
use Filament\Infolists\Components\RepeatableEntry;
RepeatableEntry::make('comments')
->schema([
// ...
])
->grid(2)
This method accepts the same options as the columns()
method of the grid. This allows you to responsively customize the number of grid columns at various breakpoints.
As well as allowing a static value, the grid()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Entry | Filament\Infolists\Components\Entry | $component | The current entry component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the entry. |
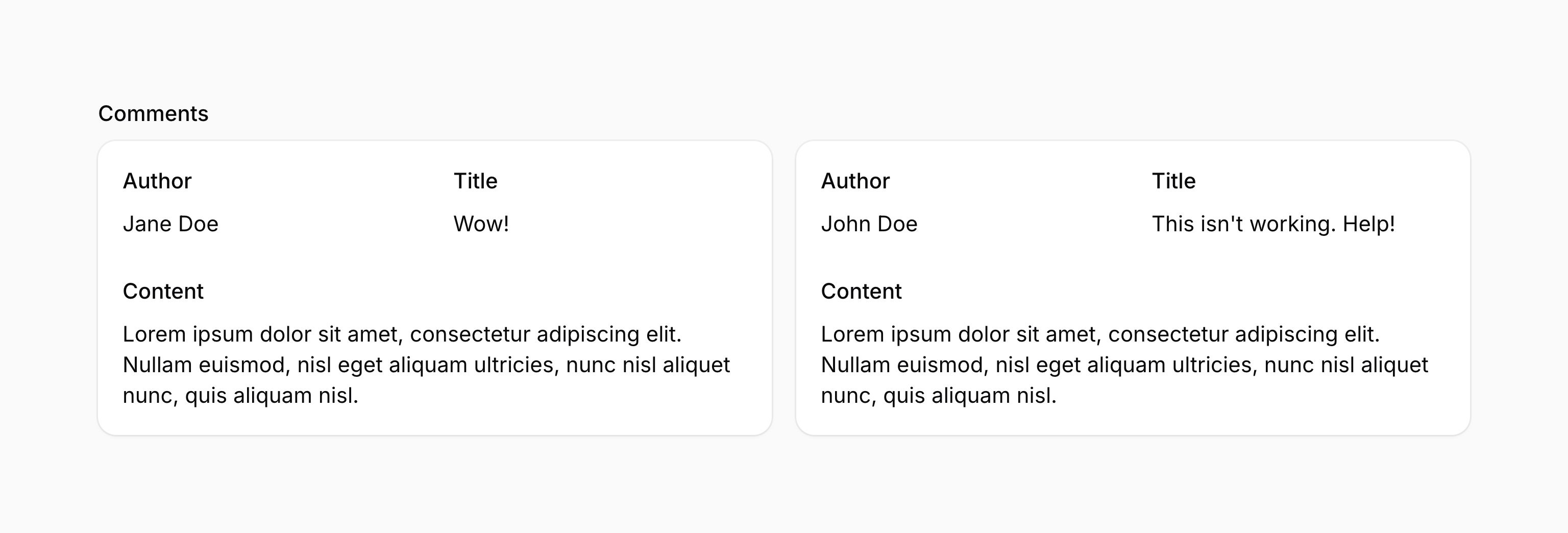
Removing the styled container
By default, each item in a repeatable entry is wrapped in a container styled as a card. You may remove the styled container using contained()
:
use Filament\Infolists\Components\RepeatableEntry;
RepeatableEntry::make('comments')
->schema([
// ...
])
->contained(false)
As well as allowing a static value, the contained()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Entry | Filament\Infolists\Components\Entry | $component | The current entry component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the entry. |
Still need help? Join our Discord community or open a GitHub discussion