Schemas
Layouts
Introduction
Filament’s grid system allows you to create responsive, multi-column layouts using any layout component. Filament provides a set of built-in layout components to help you build these:
You may also create your own custom layout components to display components however you wish.
Grid system
All layout components have a columns()
method that you can use in a couple of different ways:
- You can pass an integer like
columns(2)
. This integer is the number of columns used on thelg
breakpoint and higher. All smaller devices will have just 1 column. - You can pass an array, where the key is the breakpoint and the value is the number of columns. For example,
columns(['md' => 2, 'xl' => 4])
will create a 2 column layout on medium devices, and a 4 column layout on extra large devices. The default breakpoint for smaller devices uses 1 column, unless you use adefault
array key.
Breakpoints (sm
, md
, lg
, xl
, 2xl
) are defined by Tailwind, and can be found in the Tailwind documentation.
As well as allowing a static value, the columns()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Component | Filament\Schemas\Components\Component | $component | The current component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
Grid column spans
In addition to specifying how many columns a layout component should have, you may also specify how many columns a component should fill within the parent grid, using the columnSpan()
method. This method accepts an integer or an array of breakpoints and column spans:
columnSpan(2)
will make the component fill up to 2 columns on all breakpoints.columnSpan(['md' => 2, 'xl' => 4])
will make the component fill up to 2 columns on medium devices, and up to 4 columns on extra large devices. The default breakpoint for smaller devices uses 1 column, unless you use adefault
array key.columnSpan('full')
orcolumnSpanFull()
orcolumnSpan(['default' => 'full'])
will make the component fill the full width of the parent grid, regardless of how many columns it has.
As well as allowing a static value, the columnSpan()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Component | Filament\Schemas\Components\Component | $component | The current component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
Grid column starts
If you want to start a component in a grid at a specific column, you can use the columnStart()
method. This method accepts an integer, or an array of breakpoints and which column the component should start at:
columnStart(2)
will make the component start at column 2 on all breakpoints.columnStart(['md' => 2, 'xl' => 4])
will make the component start at column 2 on medium devices, and at column 4 on extra large devices. The default breakpoint for smaller devices uses 1 column, unless you use adefault
array key.
use Filament\Schemas\Components\Grid;
use Filament\Schemas\Components\TextEntry;
Grid::make()
->columns([
'sm' => 3,
'xl' => 6,
'2xl' => 8,
])
->schema([
TextInput::make('name')
->columnStart([
'sm' => 2,
'xl' => 3,
'2xl' => 4,
]),
// ...
])
In this example, the grid has 3 columns on small devices, 6 columns on extra large devices, and 8 columns on extra extra large devices. The text input will start at column 2 on small devices, column 3 on extra large devices, and column 4 on extra extra large devices. This is essentially producing a layout whereby the text input always starts halfway through the grid, regardless of how many columns the grid has.
As well as allowing a static value, the columnStart()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Component | Filament\Schemas\Components\Component | $component | The current component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
An example of a responsive grid layout
In this example, we have a schema with a section layout component. Since all layout components support the columns()
method, we can use it to create a responsive grid layout within the section itself.
We pass an array to columns()
as we want to specify different numbers of columns for different breakpoints. On devices smaller than the sm
Tailwind breakpoint, we want to have 1 column, which is default. On devices larger than the sm
breakpoint, we want to have 3 columns. On devices larger than the xl
breakpoint, we want to have 6 columns. On devices larger than the 2xl
breakpoint, we want to have 8 columns.
Inside the section, we have a text input. Since text inputs are form fields and all components have a columnSpan()
method, we can use it to specify how many columns the text input should fill. On devices smaller than the sm
breakpoint, we want the text input to fill 1 column, which is default. On devices larger than the sm
breakpoint, we want the text input to fill 2 columns. On devices larger than the xl
breakpoint, we want the text input to fill 3 columns. On devices larger than the 2xl
breakpoint, we want the text input to fill 4 columns.
use Filament\Forms\Components\TextInput;
use Filament\Schemas\Components\Section;
Section::make()
->columns([
'sm' => 3,
'xl' => 6,
'2xl' => 8,
])
->schema([
TextInput::make('name')
->columnSpan([
'sm' => 2,
'xl' => 3,
'2xl' => 4,
]),
// ...
])
Basic layout components
Grid component
All layout components support the columns()
method, but you also have access to an additional Grid
component. If you feel that your schema would benefit from an explicit grid syntax with no extra styling, it may be useful to you. Instead of using the columns()
method, you can pass your column configuration directly to Grid::make()
:
use Filament\Schemas\Components\Grid;
Grid::make([
'default' => 1,
'sm' => 2,
'md' => 3,
'lg' => 4,
'xl' => 6,
'2xl' => 8,
])
->schema([
// ...
])
Flex component
The Flex
component allows you to define layouts with flexible widths, using flexbox. This component does not use Filament’s grid system.
use Filament\Forms\Components\Textarea;
use Filament\Forms\Components\TextInput;
use Filament\Forms\Components\Toggle;
use Filament\Schemas\Components\Section;
use Filament\Schemas\Components\Flex;
Flex::make([
Section::make([
TextInput::make('title'),
Textarea::make('content'),
]),
Section::make([
Toggle::make('is_published'),
Toggle::make('is_featured'),
])->grow(false),
])->from('md')
In this example, the first section will grow()
to consume available horizontal space, without affecting the amount of space needed to render the second section. This creates a flexible width sidebar effect.
The from()
method is used to control the Tailwind breakpoint (sm
, md
, lg
, xl
, 2xl
) at which the horizontally-split layout should be used. In this example, the horizontally-split layout will be used on medium devices and larger. On smaller devices, the sections will stack on top of each other.
As well as allowing static values, the grow()
and from()
methods also accept functions to dynamically calculate them. You can inject various utilities into the functions as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Component | Filament\Schemas\Components\Component | $component | The current component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
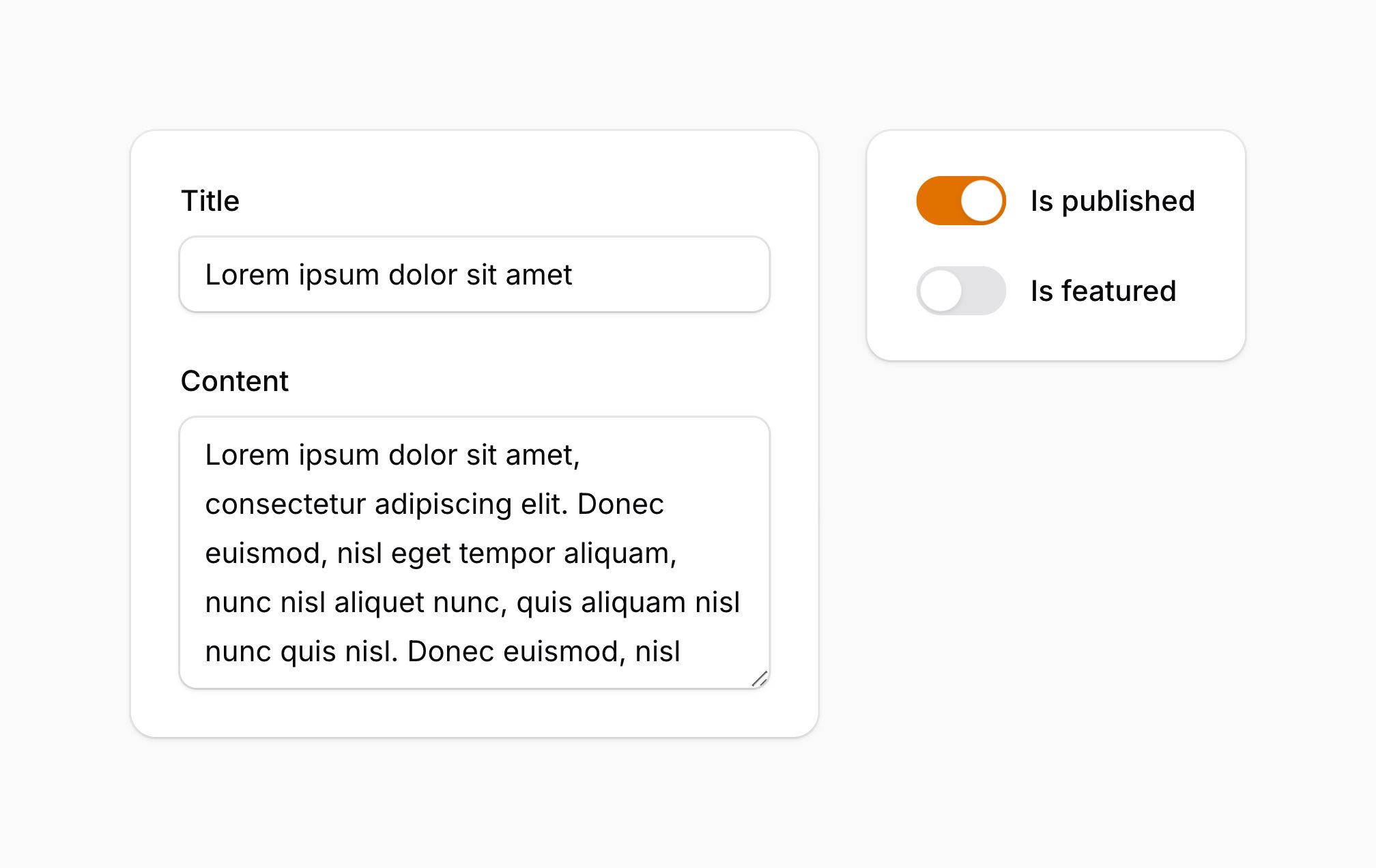
Fieldset component
You may want to group fields into a Fieldset. Each fieldset has a label, a border, and a two-column grid by default:
use Filament\Schemas\Components\Fieldset;
Fieldset::make('Label')
->columns([
'default' => 1,
'md' => 2,
'xl' => 3,
])
->schema([
// ...
])
As well as allowing a static label, the make()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Component | Filament\Schemas\Components\Component | $component | The current component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
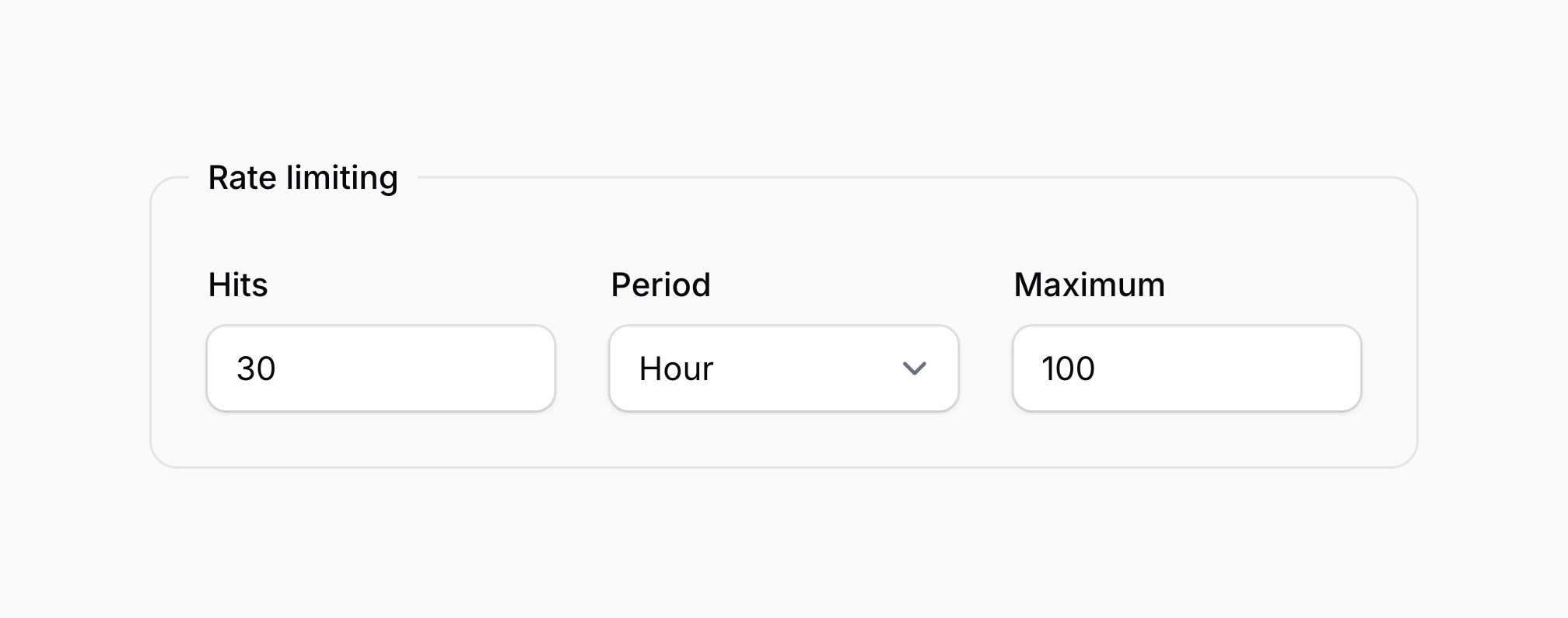
Removing the border from a fieldset
You can remove the container border from a fieldset using the contained(false)
method:
use Filament\Schemas\Components\Fieldset;
Fieldset::make('Label')
->contained(false)
->schema([
// ...
])
As well as allowing a static value, the contained()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Component | Filament\Schemas\Components\Component | $component | The current component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
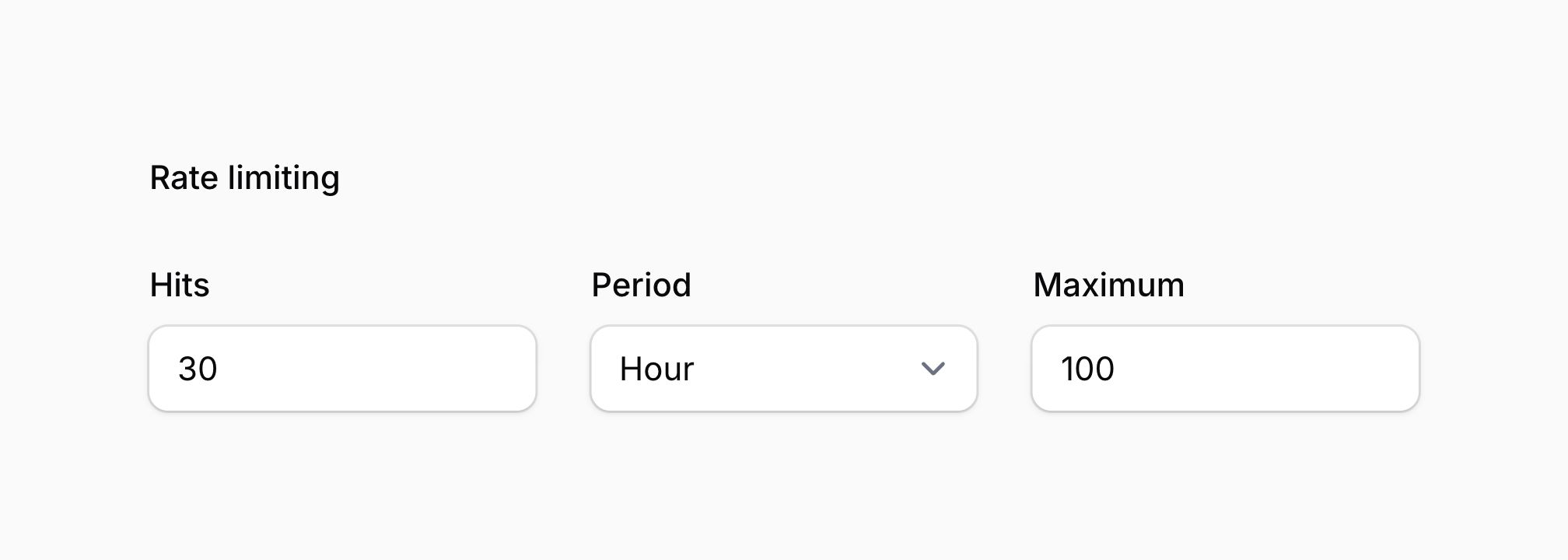
Using container queries
In addition to traditional breakpoints based on the size of the viewport, you can also use container queries to create responsive layouts based on the size of a parent container. This is particularly useful when the size of the parent container is not directly tied to the size of the viewport. For example, when using a collapsible sidebar alongside the content, the content area dynamically adjusts its size depending on the collapse state of the sidebar.
The foundation of a container query is the container itself. The container is the element whose width determines the layout. To designate an element as a container, use the gridContainer()
method on it. For instance, if you want to define the number of grid columns in a [Grid
component] based on its width:
use Filament\Schemas\Components\Grid;
Grid::make()
->gridContainer()
->columns([
// ...
])
->schema([
// ...
])
Once an element is specified as a grid container, the element or any of its children can utilize container breakpoints instead of standard breakpoints. For example, you could use @md
to define the number of grid columns when the container’s width is at least 448px
, and @xl
for when the width is at least 576px
.
use Filament\Schemas\Components\Grid;
Grid::make()
->gridContainer()
->columns([
'@md' => 3,
'@xl' => 4,
])
->schema([
// ...
])
You can also use container breakpoints in the columnSpan()
and columnStart()
methods:
use Filament\Schemas\Components\Grid;
use Filament\Schemas\Components\TextInput;
Grid::make()
->gridContainer()
->columns([
'@md' => 3,
'@xl' => 4,
])
->schema([
TextInput::make('name')
->columnSpan([
'@md' => 2,
'@xl' => 3,
]),
// ...
])
Supporting container queries on older browsers
Container queries are not yet widely supported in browsers compared to traditional breakpoints. To support older browsers, you can define an additional layer of breakpoints alongside the container breakpoints. By prefixing the traditional breakpoint with !@
, you can specify that the fallback breakpoint should be used when container queries are not supported in the browser.
For example, if you want to use the @md
container breakpoint for the grid columns but also support older browsers, you can define the !@md
fallback breakpoint, which will be applied when container queries are unavailable:
use Filament\Schemas\Components\Grid;
Grid::make()
->gridContainer()
->columns([
'@md' => 3,
'@xl' => 4,
'!@md' => 2,
'!@xl' => 3,
])
->schema([
// ...
])
You can also use !@
fallback breakpoints in the columnSpan()
and columnStart()
methods.
Adding extra HTML attributes to a layout component
You can pass extra HTML attributes to the component via the extraAttributes()
method, which will be merged onto its outer HTML element. The attributes should be represented by an array, where the key is the attribute name and the value is the attribute value:
use Filament\Schemas\Components\Section;
Section::make()
->extraAttributes(['class' => 'custom-section-style'])
As well as allowing a static value, the extraAttributes()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Component | Filament\Schemas\Components\Component | $component | The current component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
By default, calling extraAttributes()
multiple times will overwrite the previous attributes. If you wish to merge the attributes instead, you can pass merge: true
to the method.
Still need help? Join our Discord community or open a GitHub discussion