Infolists
Key-value entry
Introduction
The key-value entry allows you to render key-value pairs of data, from a one-dimensional JSON object / PHP array.
use Filament\Infolists\Components\KeyValueEntry;
KeyValueEntry::make('meta')
For example, the state of this entry might be represented as:
[
'description' => 'Filament is a collection of Laravel packages',
'og:type' => 'website',
'og:site_name' => 'Filament',
]
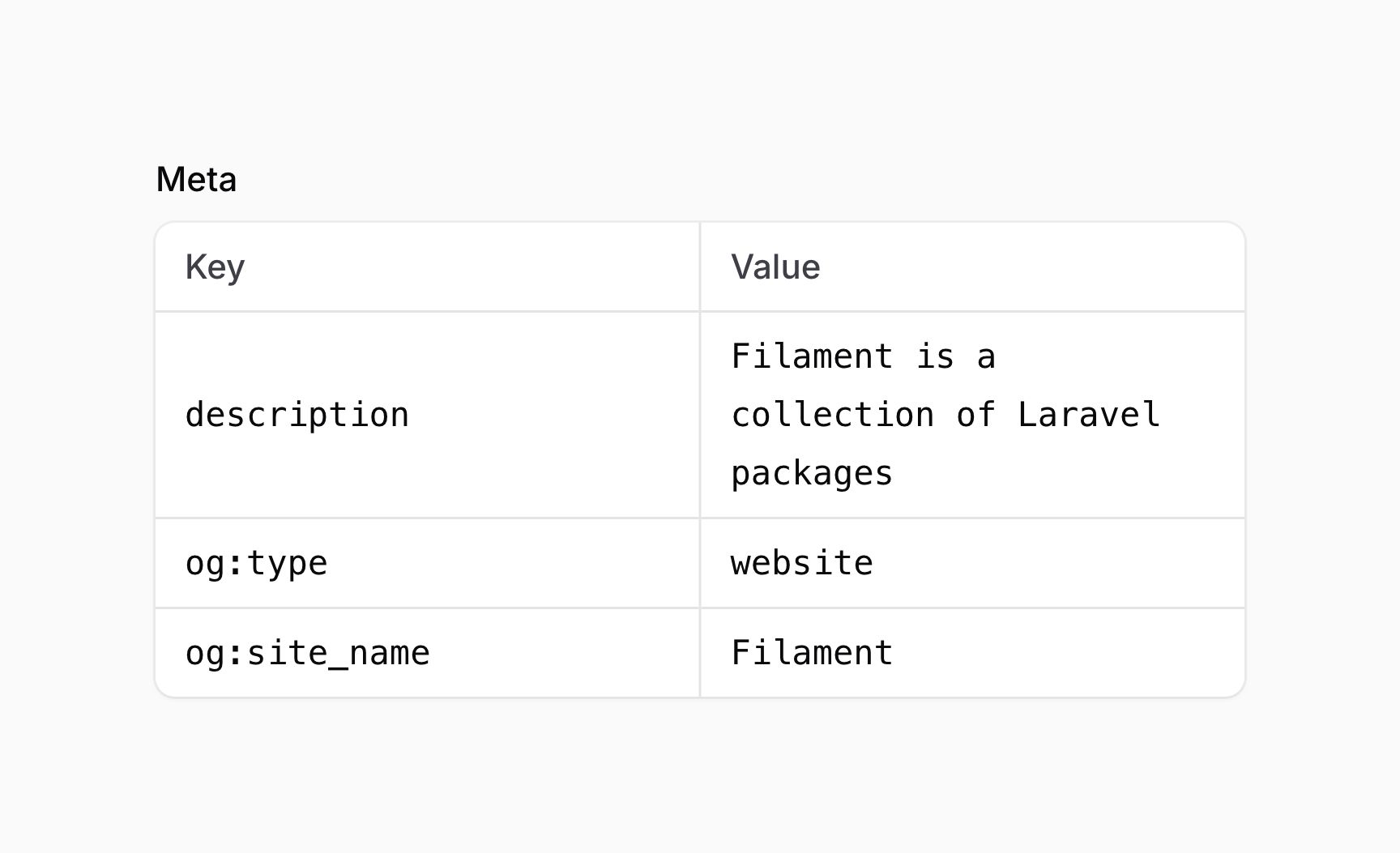
If you’re saving the data in Eloquent, you should be sure to add an array
cast to the model property:
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
protected $casts = [
'meta' => 'array',
];
// ...
}
Customizing the key column’s label
You may customize the label for the key column using the keyLabel()
method:
use Filament\Infolists\Components\KeyValueEntry;
KeyValueEntry::make('meta')
->keyLabel('Property name')
As well as allowing a static value, the keyLabel()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Entry | Filament\Infolists\Components\Entry | $component | The current entry component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the entry. |
Customizing the value column’s label
You may customize the label for the value column using the valueLabel()
method:
use Filament\Infolists\Components\KeyValueEntry;
KeyValueEntry::make('meta')
->valueLabel('Property value')
As well as allowing a static value, the valueLabel()
method also accepts a function to dynamically calculate it. You can inject various utilities into the function as parameters.
Learn more about utility injection.
Utility | Type | Parameter | Description |
---|---|---|---|
Entry | Filament\Infolists\Components\Entry | $component | The current entry component instance. |
Get function | Filament\Schemas\Components\Utilities\Get | $get | A function for retrieving values from the current schema data. Validation is not run on form fields. |
Livewire | Livewire\Component | $livewire | The Livewire component instance. |
Eloquent model FQN | ?string<Illuminate\Database\Eloquent\Model> | $model | The Eloquent model FQN for the current schema. |
Operation | string | $operation | The current operation being performed by the schema. Usually create , edit , or view . |
Eloquent record | ?Illuminate\Database\Eloquent\Model | $record | The Eloquent record for the current schema. |
State | mixed | $state | The current value of the entry. |
Still need help? Join our Discord community or open a GitHub discussion